Mondrian Art
Start: 10/24/2019
Due: 10/30/2019, by the beginning of class
Collaboration: individual
Important Link: gradesheet
This assignment was designed by Ben Stephenson, University of Calgary. Original language was Haskell, outputing SVG tags.
Overview
Piet Mondrian (1872-1944) was a Dutch painter regarded as one of the greatest artists of the 20th century. He was a pioneer in abstract art and created numerous paintings using only simple geometric elements. The site http://www.piet-mondrian.org/paintings.jsp contains a collection of his paintings. In this project you will write a program to generate art in the Mondrian style. Your program’s output should be an image drawn with the Processing library.
Specific tasks
The high-level algorithm for generating a mondrian art should work like this. Given a rectangle (to be called “region” from now on), we decide to do exactly one of the following things.
- Split the region into 4 smaller regions using a horizontal line and a vertical line. Recurse on each subregion.
- Split the region into 2 smaller regions of equal height, positioned side by side. Recurse on each subregion.
- Split the region into 2 smaller regions of equal width, one positioned on top of another. Recurse on each subregion.
- Do no splitting. Just draw the region with black outline and fill it with a random color.
Here is the algorithm in detail. We decide on one of the 4 choices above using the following algorithm:
Choose choice 1 if
(the region is wider than half the initial canvas width
and taller than half the initial canvas height)
or
(the region is big enough to be split both horizontally
and vertically and was chosen to be split, with a
probability of 2/3 along each dimension)
Choose choice 2 if
(choice 1 does not apply)
and
(the region is wider than half the initial canvas width
or
the region is wide enough to be split horizontally
and was chosen to be split, with a probability of 2/3)
Choose choice 3 if
(choice 1 and 2 do not apply)
and
(the region is taller than half the initial canvas height
or
the region is tall enough to be split vertically
and was chosen to be split, with a probability of 2/3)
Choose choice 4 if
none of the above choices 1, 2, or 3 applies
The word “big enough” in the algorithm above can be arbitrarily defined to be, say, at least 60 units.
Once a decision has been made on splitting, we proceed like this.
If we decide to split into 4 regions:
choose a random vertical line intersecting the middle one-third
of the width of the rectangle;
choose a random horizontal line intersecting the middle one-third
of the height of the rectangle;
split the region into 4 smaller regions along the chosen lines;
recursively call the mondrian function on each subregion;
else if we decide to split into 2 side-by-side regions:
choose a random vertical line intersecting the middle one-third
of the width of the rectangle;
split the region into 2 smaller regions along the chosen line;
recursively call the mondrian function on each subregion;
else if we decide to split into 2 regions, one on top of another:
choose a random horizontal line intersecting the middle one-third
of the height of the rectangle;
split the region into 2 smaller regions along the chosen line;
recursively call the mondrian function on each subregion;
else:
fill the current region (randomly, either white or colored,
with white 3 times as likely as colored, and if colored,
with a random determination of red, blue or yellow);
An image generated using this algorithm is shown below.
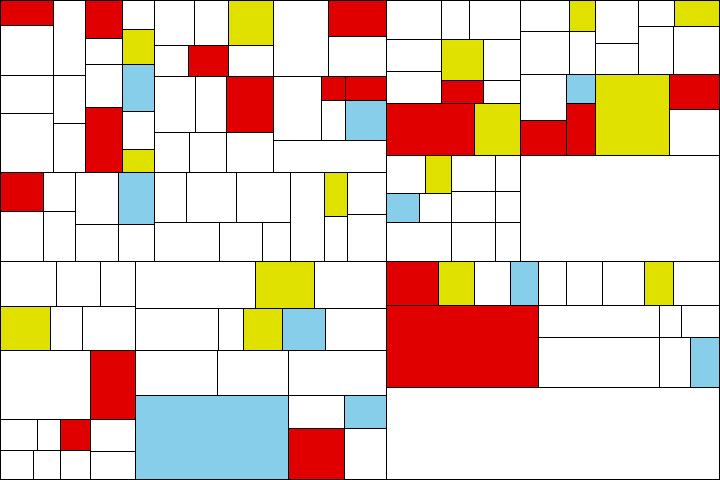
Once you have the provided algorithm working, save a copy of your program under the name MondrianBasic.kt
. You are then encouraged to adjust/expand/adapt this algorithm to generate art with your own specific style provided that it is still at least vaguely Mondrian in style (meaning that it largely consists of horizontal and vertical lines and colored regions, at least the majority of which are rectangular). Ideas for customizing your work that you might want to consider include:
- Using lines of variable width
- Using a broader color palette than red, yellow and blue for the filled regions
- Changing the distribution of random numbers used when selecting the sizes of regions, colors (or anything else random). For example:
- Instead of using numbers that are an even random distribution, you could add two random numbers together and divide by two to form a distribution that favors numbers in the middle of the random space.
- Instead of allowing all possible values, reduce the space to numbers that are evenly divisible by 10 (or 20 or some other number) so that the random lines have more regular spacing to them.
- Using a patterned fill for some regions instead of only using solid fills
- Occasionally splitting a region into something other than rectangles
- Occasionally split a region into 3 smaller regions instead of 2 or 4
Here are a couple of images for inspiration. In both of these images, the color used to fill the region is influenced (but not fully determined) by its location:
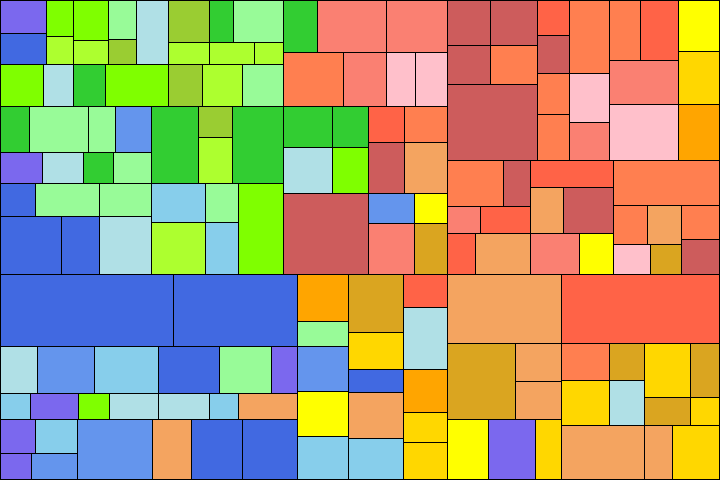
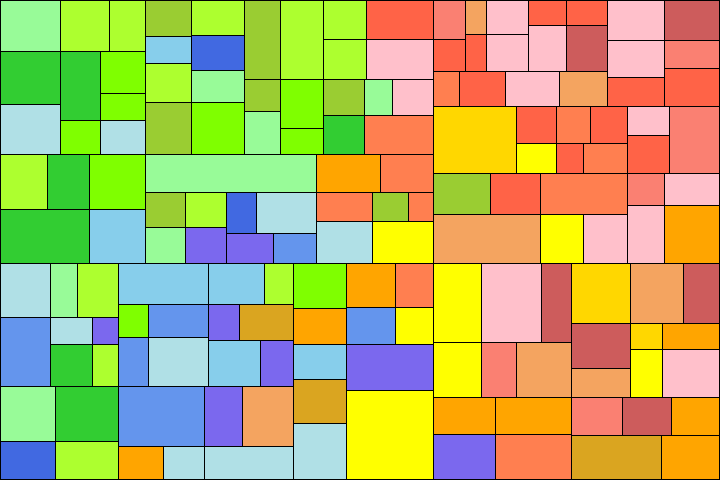
Your extended version of the program should be named MondrianExtended.kt
.
Extra Credit
The first possible extension to the programs is to make them interactive. Every time the user presses a (non-ESC) key, make the programs generate and display a new art. Once they can do that, make them respond differently to the key press event depending on what key the user presses. If s
or S
is pressed, make the programs generate a new file name and save the art work to a file with that name. If any other key is pressed, make them generate and display a new art like before. Do you see why this extension is useful?
The second extension is to write a version of your program that not only displays the art work but also animates its drawing. This version does not need to have the feature described in the previous paragraph, i.e., it doesn’t have to respond to the key press event. You can either extend from MondrianBasic.kt
to AnimatedMondrianBasic.kt
or from MondrianExtended.kt
to AnimatedMondrianExtended.kt
.
Gradesheet
We will use this gradesheet when grading your lab.
Submission
You must implement the basic algorithm described in this document and save and submit a version of your code that implements the basic algorithm. Then you are free to go on to modify/extend your program to demonstrate your artistic talents. Failure to submit a version of the program that implements the provided algorithm will cause us to assume that any difference occurred because you weren’t able to implement the algorithm correctly rather than due to artistic choice.
Since the results from your program are random, you may also want to submit a few image files for your favorite images that your programs have generated since we will probably not see the same images when we test your programs.
Submit your .kt file (or files if you are attempting A/A+ or extra credit work). See gradesheet. Zip up your files (if submitting more than 1 file) and submit via Moodle.